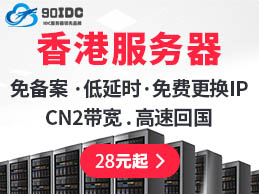
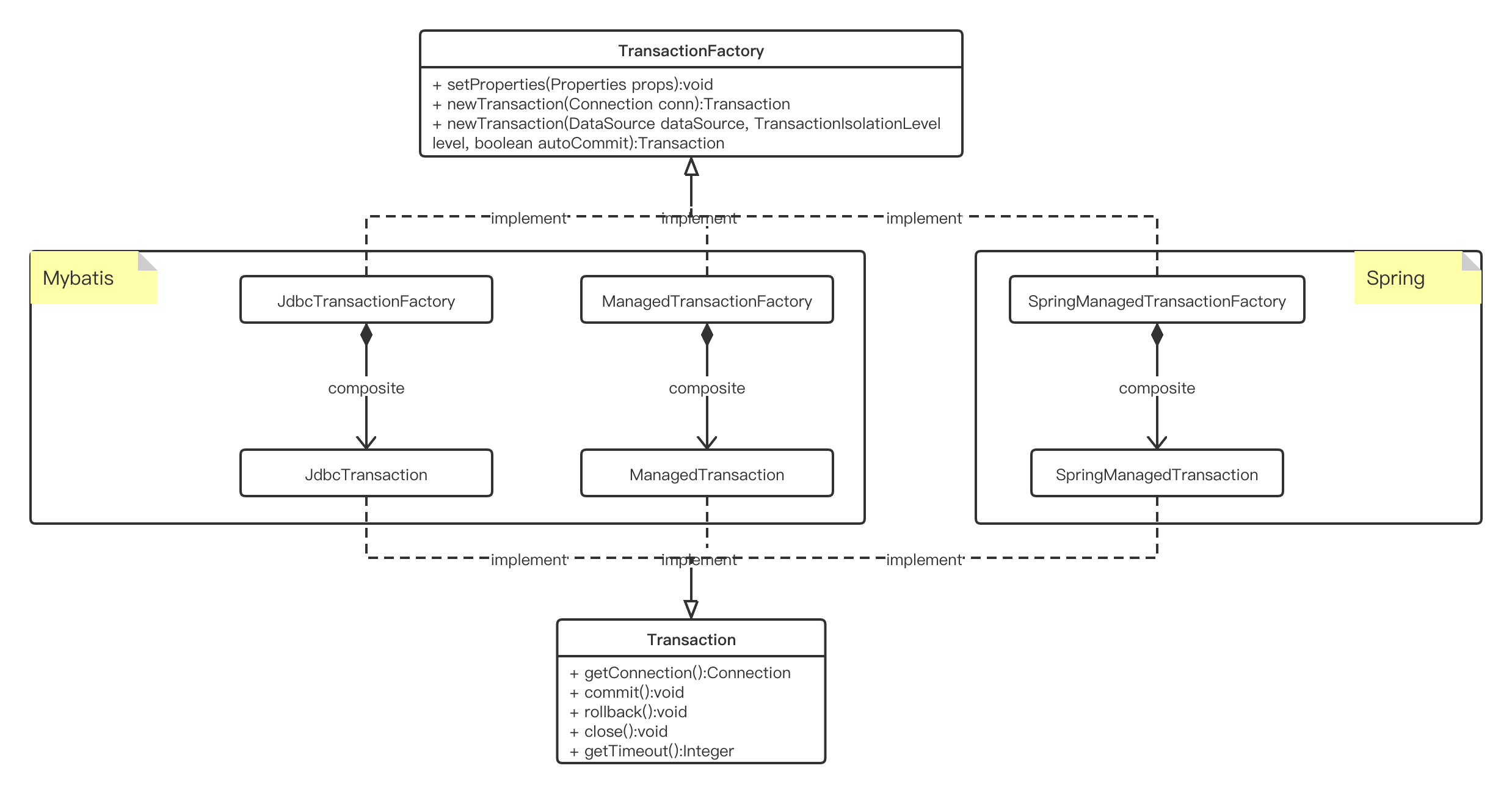
TransactionFactory
public interface TransactionFactory {
default void setProperties(Properties props) {
// NOP
}
Transaction newTransaction(Connection conn);
Transaction newTransaction(DataSource dataSource, TransactionIsolationLevel level, boolean autoCommit);
}
Transaction
public interface Transaction {
Connection getConnection() throws SQLException;
void commit() throws SQLException;
void rollback() throws SQLException;
void close() throws SQLException;
// 获取事务超时时间(Spring的SpringManagedTransaction,存在对应实现)
Integer getTimeout() throws SQLException;
}
SpringManagedTransaction
public class SpringManagedTransaction implements Transaction {
private static final Log LOGGER = LogFactory.getLog(SpringManagedTransaction.class);
private final DataSource dataSource;
private Connection connection;
// 当前连接是否为事务连接
private boolean isConnectionTransactional;
// 是否自动提交。如果是自动提交,也就不需要手动commit()了
private boolean autoCommit;
public SpringManagedTransaction(DataSource dataSource) {
Assert.notNull(dataSource, "No DataSource specified");
this.dataSource = dataSource;
}
public Connection getConnection() throws SQLException {
if (this.connection == null) {
this.openConnection();
}
return this.connection;
}
private void openConnection() throws SQLException {
this.connection = DataSourceUtils.getConnection(this.dataSource);
this.autoCommit = this.connection.getAutoCommit();
// DataSourceUtils获取对应的事务性ConnectionHolder,然后比对当前连接与ConnectionHolder
this.isConnectionTransactional = DataSourceUtils.isConnectionTransactional(this.connection, this.dataSource);
if (LOGGER.isDebugEnabled()) {
LOGGER.debug("JDBC Connection [" + this.connection + "] will" + (this.isConnectionTransactional ? " " : " not ") + "be managed by Spring");
}
}
public void commit() throws SQLException {
if (this.connection != null && !this.isConnectionTransactional && !this.autoCommit) {
if (LOGGER.isDebugEnabled()) {
LOGGER.debug("Committing JDBC Connection [" + this.connection + "]");
}
// 事务提交
this.connection.commit();
}
}
public void rollback() throws SQLException {
if (this.connection != null && !this.isConnectionTransactional && !this.autoCommit) {
if (LOGGER.isDebugEnabled()) {
LOGGER.debug("Rolling back JDBC Connection [" + this.connection + "]");
}
// 事务回滚
this.connection.rollback();
}
}
public void close() throws SQLException {
// 通过DataSourceUtils,释放当前连接。依旧涉及ConnectionHolder
DataSourceUtils.releaseConnection(this.connection, this.dataSource);
}
public Integer getTimeout() throws SQLException {
// Connection没有对应的事务超时时间,这里直接调用底层实现,获取事务超时时间
ConnectionHolder holder = (ConnectionHolder)TransactionSynchronizationManager.getResource(this.dataSource);
return holder != null && holder.hasTimeout() ? holder.getTimeToLiveInSeconds() : null;
}
}
这里的实现,涉及Connection的事务实现、DataSourceUtils、TransactionSynchronizationManager.getResource三个点。
f.缓存模块
Cache:多种实现。如FIFO、LRU
CacheKey:应对SQL的可变参数
TransactionalCacheManager&TransactionalCache:事务缓存
缓存模块,是直接关联执行模块-Executor模块
Mybatis的缓存:
一级缓存:默认开启。属于SqlSession级别的缓存。利用BaseExecute -> PerpetualCache -> HashMap<Obj,Obj>实现。
二级缓存:默认关闭。属于全局级别的缓存。利用CacheExecute -> TransactionalCacheManager -> HashMap<Cache, TransactionalCache> -> TransactionalCache
缓存实现,采用装饰器模式
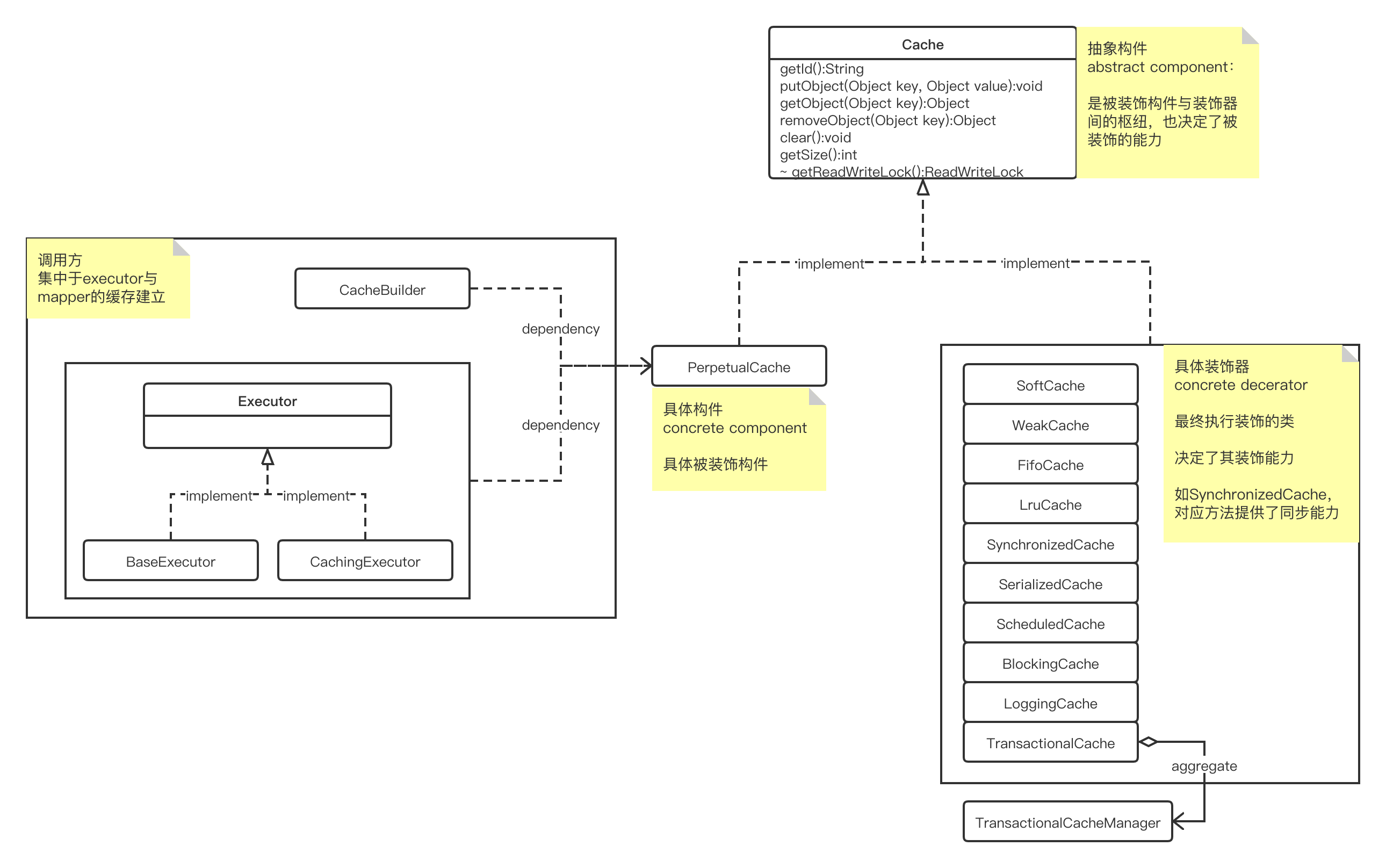