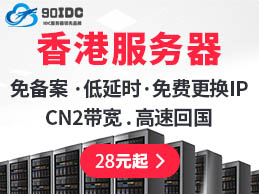

public class Blog:FullAuditedAggregateRoot<Guid>
{
[NotNull]
public virtual string Name { get; protected set; }
[NotNull]
public virtual string ShortName { get; protected set; }
[CanBeNull]
public virtual string Description { get; set; }
protected Blog()
{
}
public Blog(Guid id, [NotNull] string name, [NotNull] string shortName)
{
//属性赋值
Id = id;
//有效性检测
Name = Check.NotNullOrWhiteSpace(name, nameof(name));
//有效性检测
ShortName = Check.NotNullOrWhiteSpace(shortName, nameof(shortName));
}
public virtual Blog SetName([NotNull] string name)
{
Name = Check.NotNullOrWhiteSpace(name, nameof(name));
return this;
}
public virtual Blog SetShortName(string shortName)
{
ShortName = Check.NotNullOrWhiteSpace(shortName, nameof(shortName));
return this;
}
}
public class Comment : FullAuditedAggregateRoot<Guid>
{
public virtual Guid PostId { get; protected set; }
public virtual Guid? RepliedCommentId { get; protected set; }
public virtual string Text { get; protected set; }
protected Comment()
{
}
public Comment(Guid id, Guid postId, Guid? repliedCommentId, [NotNull] string text)
{
Id = id;
PostId = postId;
RepliedCommentId = repliedCommentId;
Text = Check.NotNullOrWhiteSpace(text, nameof(text));
}
public void SetText(string text)
{
Text = Check.NotNullOrWhiteSpace(text, nameof(text));
}
}
public class Post : FullAuditedAggregateRoot<Guid>
{
public virtual Guid BlogId { get; protected set; }
[NotNull]
public virtual string Url { get; protected set; }
[NotNull]
public virtual string CoverImage { get; set; }
[NotNull]
public virtual string Title { get; protected set; }
[CanBeNull]
public virtual string Content { get; set; }
[CanBeNull]
public virtual string Description { get; set; }
public virtual int ReadCount { get; protected set; }
public virtual Collection<PostTag> Tags { get; protected set; }
protected Post()
{
}
public Post(Guid id, Guid blogId, [NotNull] string title, [NotNull] string coverImage, [NotNull] string url)
{
Id = id;
BlogId = blogId;
Title = Check.NotNullOrWhiteSpace(title, nameof(title));
Url = Check.NotNullOrWhiteSpace(url, nameof(url));
CoverImage = Check.NotNullOrWhiteSpace(coverImage, nameof(coverImage));
Tags = new Collection<PostTag>();
Comments = new Collection<Comment>();
}
public virtual Post IncreaseReadCount()
{
ReadCount++;
return this;
}
public virtual Post SetTitle([NotNull] string title)
{
Title = Check.NotNullOrWhiteSpace(title, nameof(title));
return this;
}
public virtual Post SetUrl([NotNull] string url)
{
Url = Check.NotNullOrWhiteSpace(url, nameof(url));
return this;
}
public virtual void AddTag(Guid tagId)
{
Tags.Add(new PostTag(Id, tagId));
}
public virtual void RemoveTag(Guid tagId)
{
Tags.RemoveAll(t => t.TagId == tagId);
}
}
public record PostTag
{
public virtual Guid TagId { get; init; } //主键
protected PostTag()
{
}
public PostTag( Guid tagId)
{
TagId = tagId;
}
}
public class Tag : FullAuditedAggregateRoot<Guid>
{
public virtual Guid BlogId { get; protected set; }
public virtual string Name { get; protected set; }
public virtual string Description { get; protected set; }
public virtual int UsageCount { get; protected internal set; }
protected Tag()
{
}
public Tag(Guid id, Guid blogId, [NotNull] string name, int usageCount = 0, string description = null)
{
Id = id;
Name = Check.NotNullOrWhiteSpace(name, nameof(name));
BlogId = blogId;
Description = description;
UsageCount = usageCount;
}
public virtual void SetName(string name)
{
Name = Check.NotNullOrWhiteSpace(name, nameof(name));
}
public virtual void IncreaseUsageCount(int number = 1)
{
UsageCount += number;
}
public virtual void DecreaseUsageCount(int number = 1)
{
if (UsageCount <= 0)
{
return;
}
if (UsageCount - number <= 0)
{
UsageCount = 0;
return;
}
UsageCount -= number;
}
public virtual void SetDescription(string description)
{
Description = description;
}
}
结语
本节知识点:
1.根据脑图划分聚合
2.根据领域图在遵守DDD的聚合根规范的情况下创建聚合